
Retrieving Fabric Tenant Settings interactively using Powershell
- data
- 2024-08-02
- 4 minutes to read
- admin
- fabric
- powershell
Table of Contents
A long time ago in a galaxy far, far away…
This post is over 3 months old, Fabric is evolving at such a rapid pace!! Please be mindful of that when reading this post, young Padawan, as it could be outdated. I try to keep things up to date as much as possible. If you think something needs updating, please let me know in the comments.
Introduction
I have been exploring Fabric APIs recently, and wanted to look into how to capture the tenant settings using simple APIs calls. This post will show how to use PowerShell to retrieve the tenant settings interactively.
Prerequisites
In order to retrieve the tenant settings, you will need to have admin rights to your Fabric tenant.
TL;DR show me the script
Here is the script in full:
$resourceUrl = 'https://api.fabric.microsoft.com'
Connect-AzAccount
$accessToken = (Get-AzAccessToken -ResourceUrl $resourceUrl).Token
$requestUrl = 'https://api.fabric.microsoft.com/v1/admin/tenantsettings'
$contentType = "application/json; charset=utf-8"
$headers = @{
'Content-Type' = $contentType
'Authorization' = "Bearer $($accessToken)"
}
((Invoke-WebRequest -Headers $headers -Method "Get" -Uri $requestUrl -Body $body -TimeoutSec 240).Content | ConvertFrom-Json).tenantSettings
How the script works
First, the Connect-AzAccount cmdlet will connect to Azure, this will prompt you to authenticate using your Azure AD credentials, you need to authenticate with an account that has permissions to the tenant settings. Once connected, the Get-AzAccessToken cmdlet is used to retrieve the access token for the Fabric API.
$resourceUrl = 'https://api.fabric.microsoft.com'
Connect-AzAccount
$accessToken = (Get-AzAccessToken -ResourceUrl $resourceUrl).Token
Next, I construct the request for the tenant settings API. The request URL is the API endpoint for retrieving the tenant settings. The headers include the content type and the access token.
$requestUrl = 'https://api.fabric.microsoft.com/v1/admin/tenantsettings'
$contentType = "application/json; charset=utf-8"
$headers = @{
'Content-Type' = $contentType
'Authorization' = "Bearer $($accessToken)"
}
And then finally I make the request to the API using the Invoke-WebRequest cmdlet. The response is then converted from JSON to a PowerShell object, and the tenant settings are extracted.
((Invoke-WebRequest -Headers $headers -Method "Get" -Uri $requestUrl -Body $body -TimeoutSec 240).Content | ConvertFrom-Json).tenantSettings
Here is a subset of the results:
settingName : FabricFeedbackTenantSwitch
title : Product Feedback
enabled : True
canSpecifySecurityGroups : False
tenantSettingGroup : Microsoft Fabric
settingName : OneLakeForThirdParty
title : Users can access data stored in OneLake with apps external to Fabric
enabled : True
canSpecifySecurityGroups : False
tenantSettingGroup : OneLake settings
settingName : OneLakeFileExplorer
title : Users can sync data in OneLake with the OneLake File Explorer app
enabled : True
canSpecifySecurityGroups : False
tenantSettingGroup : OneLake settings
settingName : QnaFeedbackLoop
title : Review questions
enabled : True
canSpecifySecurityGroups : True
tenantSettingGroup : Q&A settings
Pretty neat! This is just a snippet of the output, all tenant settings will be returned. I can either run this to observe the settings, or I can pipe the output to a file for further analysis, or tracking changes over time.
Working with the output
The output is quite long, supposing I want to look at a specific setting group, I can capture the output and then filter it using the Where-Object
cmdlet:
$tenantSettings = ((Invoke-WebRequest -Headers $headers -Method "Get" -Uri $requestUrl -Body $body -TimeoutSec 240).Content | ConvertFrom-Json).tenantSettings
$tenantSettings | Where-Object { $_.tenantSettingGroup -eq 'Export and sharing settings' }
This will return only the settings in the ‘Export and sharing settings’ group. Here is a subset of that output:
settingName : AllowExternalDataSharingSwitch
title : External data sharing (preview)
enabled : False
canSpecifySecurityGroups : True
tenantSettingGroup : Export and sharing settings
settingName : AllowExternalDataSharingReceiverSwitch
title : Users can accept external data shares (preview)
enabled : False
canSpecifySecurityGroups : True
tenantSettingGroup : Export and sharing settings
settingName : AllowGuestUserToAccessSharedContent
title : Guest users can access Microsoft Fabric
enabled : True
canSpecifySecurityGroups : True
tenantSettingGroup : Export and sharing settings
Or perhaps I want to search for all API settings. Again, I can use the Where-Object
cmdlet to filter the output. Because I have already captured the settings in an object called $tenantSettings, I can filter that object using the Where-Object
cmdlet:
$tenantSettings | Where-Object { $_.settingName -like '*API*' }
Here is the output from that command:
settingName : AllowServicePrincipalsUseReadAdminAPIs
title : Service principals can access read-only admin APIs
enabled : True
canSpecifySecurityGroups : True
enabledSecurityGroups : {@{graphId=a86b5cb8-xxxx-xxxx-xxxx-da7e98a6cfc8; name=fabric-admin-sp}, @{graphId=aca9df4c-xxxx-xxxx-xxxx-c1025546396d; name=fabric-api}}
tenantSettingGroup : Admin API settings
settingName : AllowServicePrincipalsUseWriteAdminAPIs
title : Service principals can access admin APIs used for updates
enabled : True
canSpecifySecurityGroups : True
enabledSecurityGroups : {@{graphId=aca9df4c-xxxx-xxxx-xxxx-c1025546396d; name=fabric-api}}
tenantSettingGroup : Admin API settings
settingName : AdminApisIncludeDetailedMetadata
title : Enhance admin APIs responses with detailed metadata
enabled : True
canSpecifySecurityGroups : True
enabledSecurityGroups : {@{graphId=aca9df4c-xxxx-xxxx-xxxx-c1025546396d; name=fabric-api}}
tenantSettingGroup : Admin API settings
settingName : AdminApisIncludeExpressions
title : Enhance admin APIs responses with DAX and mashup expressions
enabled : False
canSpecifySecurityGroups : True
tenantSettingGroup : Admin API settings
Wrapping Up
It’s relatively easy to set up and retrieve the tenant settings using PowerShell to evaluate the settings, but ideally we don’t want to be running this script interactively. In the next post, I will show how to run this script as a service principal, which is useful for automating the retrieval of tenant settings.
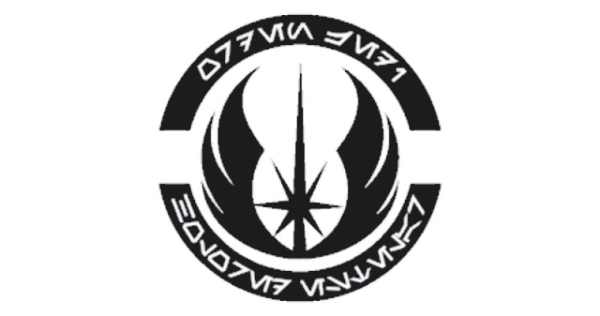
#mtfbwy